You are given an n x n 2D matrix
representing an image, rotate the image by 90 degrees (clockwise).
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1:
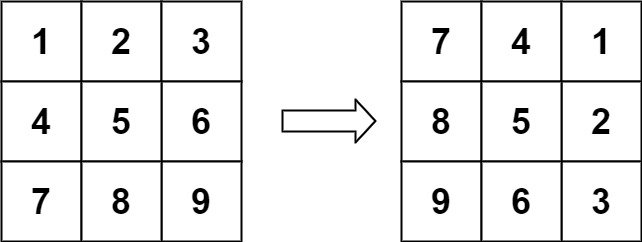
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [[7,4,1],[8,5,2],[9,6,3]]
Code
class Solution {
public:
void rotate(vector<vector<int>>& mat) {
int n = mat.size();
for(int i = 0; i < n/2; i++)
{
for(int j = i; j < n-i-1; j++)
{
int t = mat[i][j];
mat[i][j] = mat[n-1-j][i];
mat[n-1-j][i] = mat[n-1-i][n-1-j];
mat[n-1-i][n-1-j] = mat[j][n-1-i];
mat[j][n-1-i] = t;
}
}
}
};
0 Comments