Statement - Given an m x n
matrix. If an element is 0, set its entire row and column to 0. Do it in-place.
Example 1:
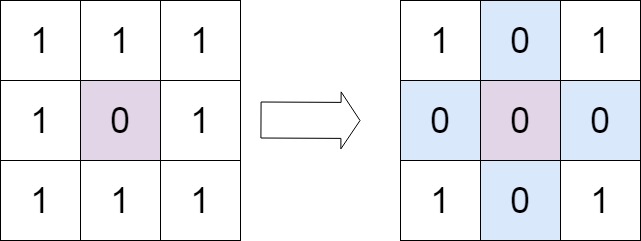
Input: matrix = [[1,1,1],[1,0,1],[1,1,1]]
Output: [[1,0,1],[0,0,0],[1,0,1]]
Problem Link - LeetCode GFG InterviewBit
Code - Time Complexity O(n^2) and Space Complexity O(1).
class Solution {
public:
void setZeroes(vector<vector<int>>& matrix) {
int row = matrix.size();
int col = matrix[0].size();
bool row0 = false, col0 = false;
for(int i = 0; i < row; i++)
{
for(int j = 0; j < col; j++)
{
if(matrix[i][j] == 0)
{
if(i == 0) row0 = true;
if(j == 0) col0 =true;
matrix[0][j] = 0;
matrix[i][0] = 0;
}
}
}
for(int i = 1; i < row; i++)
{
for(int j = 1; j < col; j++)
{
if(matrix[i][0] == 0 || matrix[0][j] == 0)
matrix[i][j] = 0;
}
}
if(row0)
{
for(int i = 0; i < col; i++)
matrix[0][i] = 0;
}
if(col0)
{
for(int i = 0; i < row; i++)
matrix[i][0] = 0;
}
}
};
0 Comments